There are multiple ways to validate IP address using PowerShell. One of them is to use regex.
The regex itself is pretty much far from being lightweight. So we end up with this:
^([01]?\d\d?|2[0-4]\d|25[0-5])\.([01]?\d\d?|2[0-4]\d|25[0-5])\.([01]?\d\d?|2[0-4]\d|25[0-5])\.([01]?\d\d?|2[0-4]\d|25[0-5])$
The safest way to get it in the console is either copy it from a separate file or type regex for one octet after another and add them together:

Although it works well, it is not the way that fits my preference.
There is another one. We can use ipaddress class and parse method. It looks as follows:
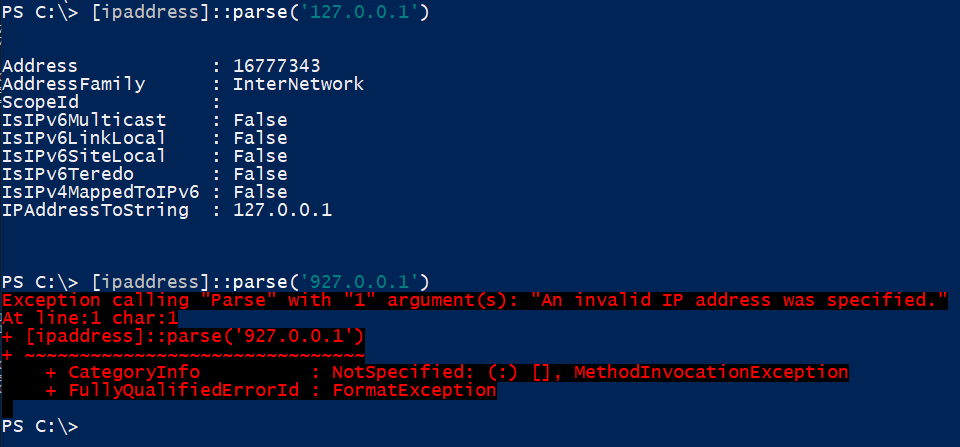
In case parsing is successful it returns a nice IP address object. There is another problem with this approach however. If we do something like this:
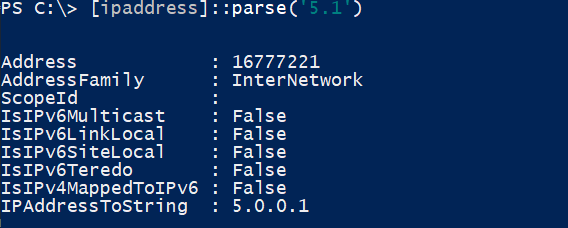
Or:
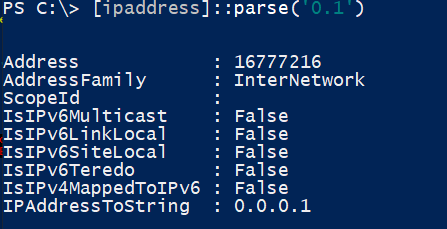
We get a parsed IP address anyway.
Although it might appear for some as a reasonable way to deal with parsing strings I would personally prefer to get an error and parse only when I have all four octets defined.
Looking for a quick way to handle this differently I ended up with a quick and funny filter:
Filter Test-IPAddress{
PROCESS{
IF(($_ -split '\.' ).count -eq 4){$IP = $_}
IF($IP -eq (( $IP | ForEach-Object{$_ -split "\."} | ForEach-Object{ IF($_ -in @(0..255)){$_}} ) -join '.' )){$IP}
}
}

Now I can pipe an array of strings and filter out the wrong IP addresses.
